Add Contact View Model in Project
- Open existing Solution in Visual Studio 2015.
- Now add a new class ContactListVM.cs in Models\Contact folder in WebApplicationCore.NetCore.
- Add required properties in ContactListVM.
- It is noticeable that we have added only those fields which are required for list view. This not only make application lighter and faster but also make application secure.
public class ContactListVM
{
[Display(Name = "Contact Id")]
public int ContactId { get; set; }
[Display(Name = "Contact Name")]
public string Name { get; set; }
[Display(Name = "Contact Number")]
public string ContactNumber { get; set; }
[Display(Name = "Email")]
public string Email { get; set; }
[Display(Name = "Web Site")]
public string WebSite { get; set; }
}

Add Contact Index View
- Add new View to Contact\Contact folder.
- Open Add New Item Screen through Solution Context Menu of Contact >> Add >> New Item >> Installed >> .NET Core >> MVC View Page.
- Name it Index.cshtml.
- Click OK Button.
- It will add a new view in Contact view folder.
- Now, we have List of ContactListVM objects as model.
- Here we have used foreach loop in view to iterate on List of Contacts.
- Add a link in GetContact View to return to Contact List page. We have explicitly omitted controller details and added action method name only. In such case action method form current controller is referenced.
- Change Index view implementation.
- Remove extra menu items not required from _Layout.cshtml. And add Menu Item for Contact List.
@model List<ContactListVM>
<h2>Contact List</h2>
<table class="table">
<thead>
<tr>
<th>
<label asp-for="FirstOrDefault().Name"></label>
</th>
<th>
<label asp-for="FirstOrDefault().ContactNumber"></label>
</th>
<th>
<label asp-for="FirstOrDefault().Email"></label>
</th>
<th>
<label asp-for="FirstOrDefault().WebSite"></label>
</th>
<th></th>
</tr>
</thead>
<tbody>
@foreach (var item in Model)
{
<tr>
<td>
<span>@item.Name</span>
</td>
<td>
<span>@item.ContactNumber</span>
</td>
<td>
<span>@item.Email</span>
</td>
<td>
<span>@item.WebSite</span>
</td>
<td>
<a asp-controller="Contact" asp-action="GetContact" asp-route-id="@item.ContactId">Get Details</a>
</td>
</tr>
}
</tbody>
</table>

Update Controller and Business Logic
- Add new method GetContacts to get all contact in WebApplicationCore.NetCore.BusinessLogic.ContactBusinessLogic class.
- Update Index Method of Contact Controller Class to load and all contact and to transform them into contactListVM.
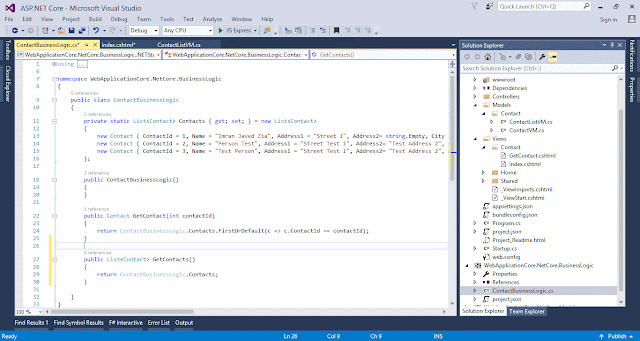
Run Application in Debug Mode
- Press F5 or Debug Menu >> Start Debugging or Start IIS Express Button on Toolbar to start application in debugging mode.
- It will show Home Page in browser.
- Click Contact List Menu Open to open Contact List Page.
- Click Get Details link of first contact and it will take us to contact details page and URL will change to http://localhost:21840/Contact/GetContact/1.
Sample Source Code
We have placed sample code for this session in "CRUD operations in ASP.NET Core 1.0 MVC Application Part 3_Code.zip" in https://aspdotnetcore.codeplex.com/SourceControl/latest CodePlex repository.
CRUD operations in ASP.NET Core 1.0 MVC Application Part 2
CRUD operations in ASP.NET Core 1.0 MVC Application Part 3
CRUD operations in ASP.NET Core 1.0 MVC Application Part 4
CRUD operations in ASP.NET Core 1.0 MVC Application Part 5
CRUD operations in ASP.NET Core 1.0 MVC Application Part 6
CRUD operations in ASP.NET Core 1.0 MVC Application Part 7
CRUD operations in ASP.NET Core 1.0 MVC Application Part 8
CRUD Operations in AP.NET Core 1.0 All Parts
CRUD operations in ASP.NET Core 1.0 MVC Application Part 1CRUD operations in ASP.NET Core 1.0 MVC Application Part 2
CRUD operations in ASP.NET Core 1.0 MVC Application Part 3
CRUD operations in ASP.NET Core 1.0 MVC Application Part 4
CRUD operations in ASP.NET Core 1.0 MVC Application Part 5
CRUD operations in ASP.NET Core 1.0 MVC Application Part 6
CRUD operations in ASP.NET Core 1.0 MVC Application Part 7
CRUD operations in ASP.NET Core 1.0 MVC Application Part 8
No comments:
Post a Comment